WEB technologies
According to Microsoft, web technologies include the following: Mark-up languages, such as HTML and CSS; Programming languages; Web server and server technologies; Databases, and Business applications.
HTML
HTML (Hyper Text Markup Language) is the code that is used to structure a web page and its content.
HTML is the standard markup language for documents designed to be displayed in a web browser. It can be assisted by such technologies as Cascading Style Sheets (CSS) and such scripting languages as JavaScript.
HTML consists of a series of elements, which you use to enclose, or wrap, different parts of the content to make it appear in a certain way, or act in a certain way.
The main parts of the element are the following:
- The opening tag: It consists of the name of the element (in this case, p), wrapped in opening and closing angle brackets. It states where the element begins or starts giving a certain effect — in this case where the paragraph begins.
- The closing tag: It is the same as the opening tag, except that it includes a forward slash before the element name. It states where the element ends — in this case where the paragraph ends. Failing to add a closing tag is one of the standard beginner errors and can lead to strange results.
- The content: It is the content of the element, which in this case, is just the text.
- The element: The opening tag, the closing tag, and the content together comprise the element.
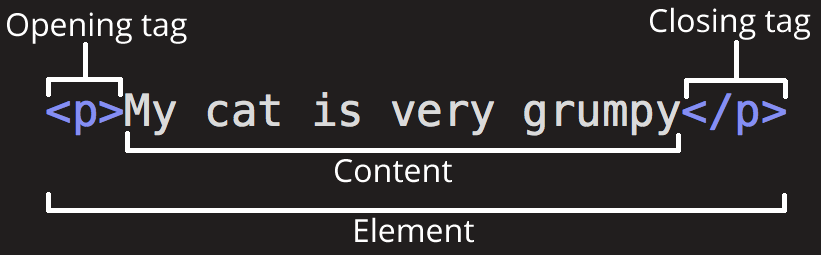
Elements can also have attributes that look like the following:

Attributes
contain extra information about the element that you don't want to appear in the
actual content. Here, class
is the attribute name and
editor-note
is the attribute value. The
class
attribute allows you to give the element a non-unique
identifier that can be used to target it (and any other elements with the same
class
value) with style information and other things.
An attribute should always have the following:
- A space between it and the element name (or the previous attribute, if the element already has one or more attributes).
- The attribute name followed by an equal sign.
- The attribute value wrapped by opening and closing quotation marks.
Anatomy of an HTML document
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>My first page</title>
</head>
<body>
<img src="images/Vistula_logo.png" alt="Vistula">
</body>
</html>
Here, we have the following:
<!DOCTYPE html>
— the doctype. It is a required preamble, The Document Type Declaration. If a declaration is not included, various browsers will revert to “quirks mode” for rendering.<html></html>
— the<html>
element. This element wraps all the content on the entire page and is sometimes known as the root element.<head></head>
— the<head>
element. This element acts as a container for all the stuff you want to include on the HTML page that isn't the content you are showing to your page's viewers.<meta charset="utf-8">
— This element sets the character set your document should use to UTF-8 which includes most characters from the vast majority of written languages.<title></title>
— the<title>
element. This element sets the title of your page, which is the title that appears in the browser tab the page is loaded in.<body></body>
— the<body>
element. It contains all the content that you want to show to web users when they visit your page, whether that's text, images, videos, games, playable audio tracks, or whatever else.
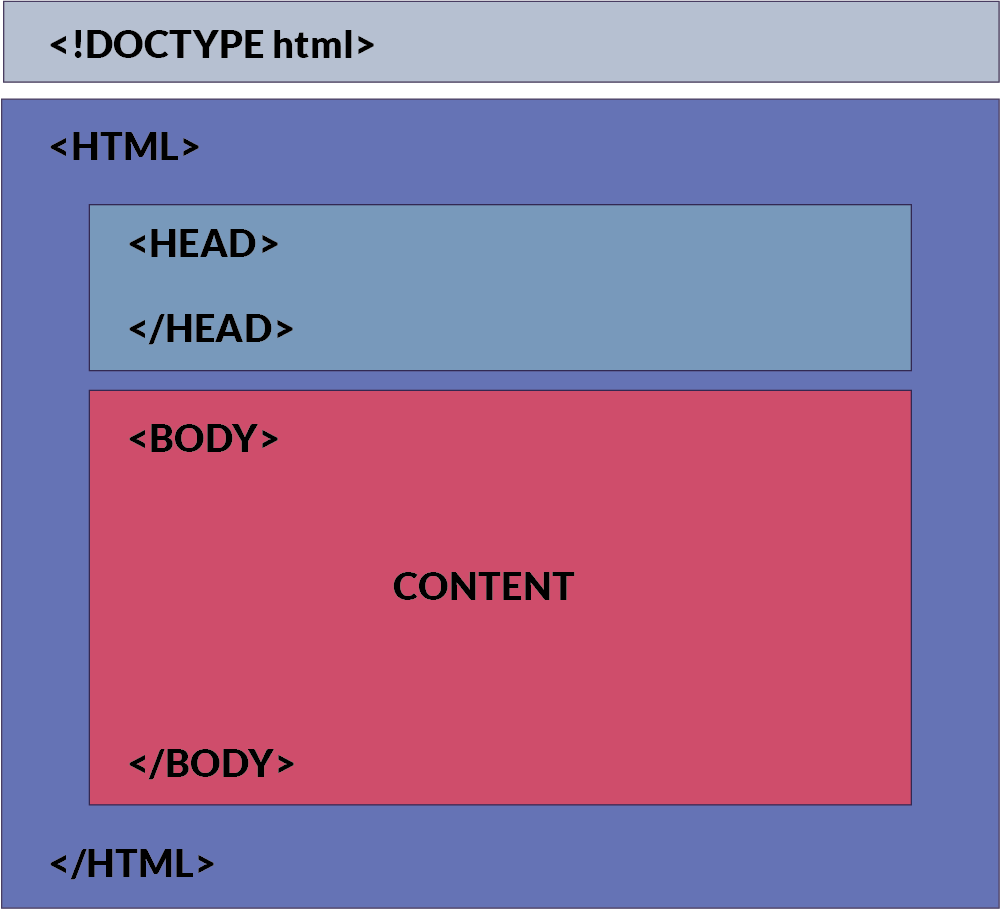
Basic body elements
- HeadingsHeading elements allow you to specify that certain parts of your content are headings — or subheadings. HTML contains 6 heading levels,
<h1>
–<h6>
.<h1>My main title</h1> <h2>My top level heading</h2> <h3>My subheading</h3> <h4>My sub-subheading</h4> <h5>My minor title</h5> <h6>My insignificant title</h6>
- Paragraphs
<p>
elements are for containing paragraphs of text.<p>This is a single paragraph</p>
- Lists
The most common list types are ordered and unordered lists:
- Unordered lists are for the lists where the order of the items
doesn't matter, such as a shopping list. These are wrapped in a
<ul>
element.<ul> <li>Milk</li> <li>Cheese <ul> <li>Blue cheese</li> <li>Feta</li> </ul> </li> </ul>
- Ordered lists are for the lists where the order of the items
matters, such as a recipe. These items are wrapped in an
<ol>
element.<ol> <li>Mix flour, baking powder, sugar, and salt.</li> <li>In another bowl, mix eggs, milk, and oil.</li> <li>Stir both mixtures together.</li> <li>Fill muffin tray 3/4 full.</li> <li>Bake for 20 minutes.</li> </ol>
Each item inside the lists is put inside a
<li>
(list item) element. - Unordered lists are for the lists where the order of the items
doesn't matter, such as a shopping list. These are wrapped in a
- Links
To add a link, use a simple element —
<a>
— "a" means a short form for "anchor".<a href="https://techwritersblog.com/">Technical writers blog</a>
- Images
The usage of the
<img>
element:- The
src
attribute is required, and contains the path to the image you want to embed. - The
alt
attribute holds a text description of the image, which isn't mandatory but is incredibly useful for accessibility — screen readers read this description out to their users so they know what the image means.
<img src="images/Vistula_logo.png" alt="Vistula">
- The
CSS
Cascading Style Sheets (CSS) is a style sheet language used for describing the presentation of a document written in a markup language such as HTML. CSS is a cornerstone technology of the World Wide Web, alongside HTML and JavaScript.
CSS is designed to enable the separation of presentation and content, including layout, colors, and fonts. This separation can improve content accessibility, provide more flexibility and control in the specification of presentation characteristics, enable multiple web pages to share formatting by specifying the relevant CSS in a separate .css file which reduces complexity and repetition in the structural content as well as enabling the .css file to be cached to improve the page load speed between the pages that share the file and its formatting.
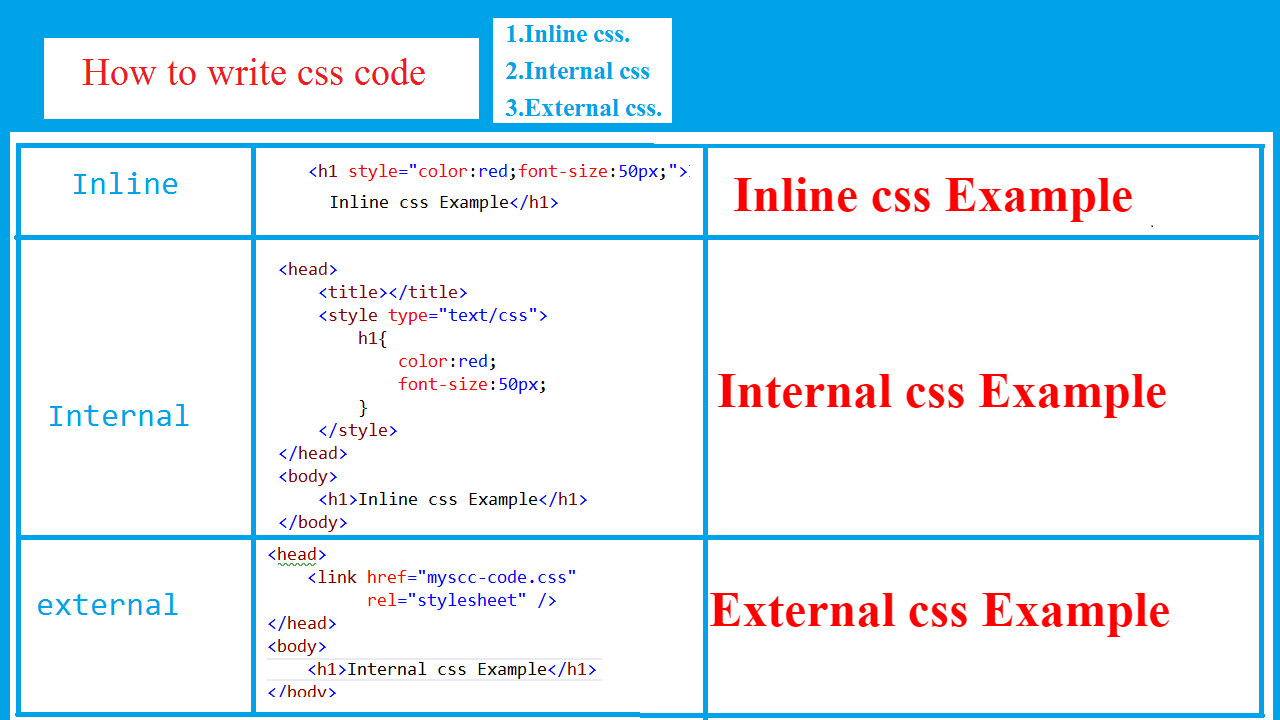
Separation of formatting and content also makes it feasible to present the same markup page in different styles for different rendering methods.
The name cascading comes from the specified priority scheme to determine which style rule applies if more than one rule matches a particular element. This cascading priority scheme is predictable.
CSS has a simple syntax and uses a number of English keywords to specify the names of various style properties.
A style sheet consists of a list of rules. Each rule or rule-set consists of one or more selectors, and a declaration block.
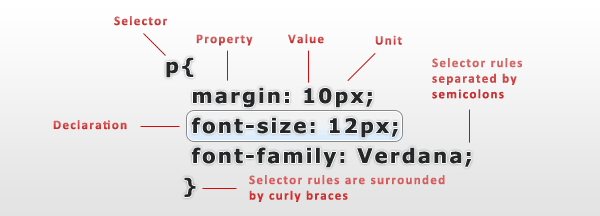
In CSS selectors declare which part of the markup a style applies to by matching tags and attributes in the markup itself.
Selectors may apply to the following:
- all elements of a specific type, for example the second-level headers h2
- elements specified by an attribute, in particular:
- id: a unique identifier within the document, identified with a hash prefix #id
- class: an identifier that can annotate multiple elements in a document, identified with a period prefix .classname
- elements depending on how they are placed relatively to others in the document tree.
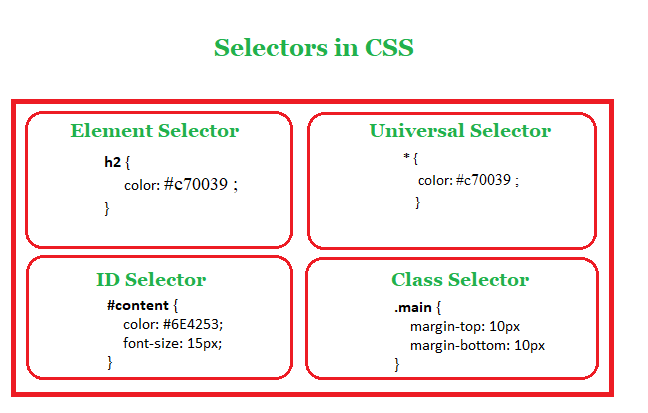
Classes and IDs are case-sensitive, start with letters, and can include alphanumeric characters, hyphens, and underscores. A class may apply to any number of instances of any elements. An ID may only be applied to a single element.
A declaration block consists of a list of declarations in braces. Each declaration itself consists of a property, a colon (:), and a value. If there are multiple declarations in a block, a semi-colon (;) must be inserted to separate each declaration. An optional semi-colon after the last (or single) declaration may be used.
Properties are specified in the CSS standard. Each property has a set of possible values. Some properties can affect any type of element, and others apply only to particular groups of elements.
Values may be keywords, such as “center” or “inherit”, or numerical values, such as 200px (200 pixels). Color values can be specified with keywords (for example, “red”), hexadecimal values (for example, #FF0000, also abbreviated as #F00), RGB values on a 0 to 255 scale (for example, rgb(255, 0, 0)), RGBA values that specify both color and alpha transparency (for example, rgba(255, 0, 0, 0.8)), or HSL or HSLA values (for example, hsl(000, 100%, 50%), hsla(000, 100%, 50%, 80%)).
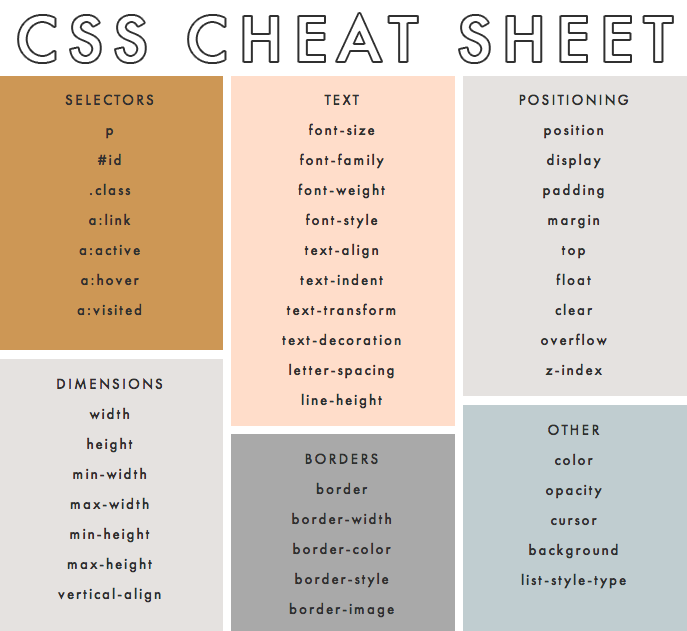
Difference between HTML and CSS
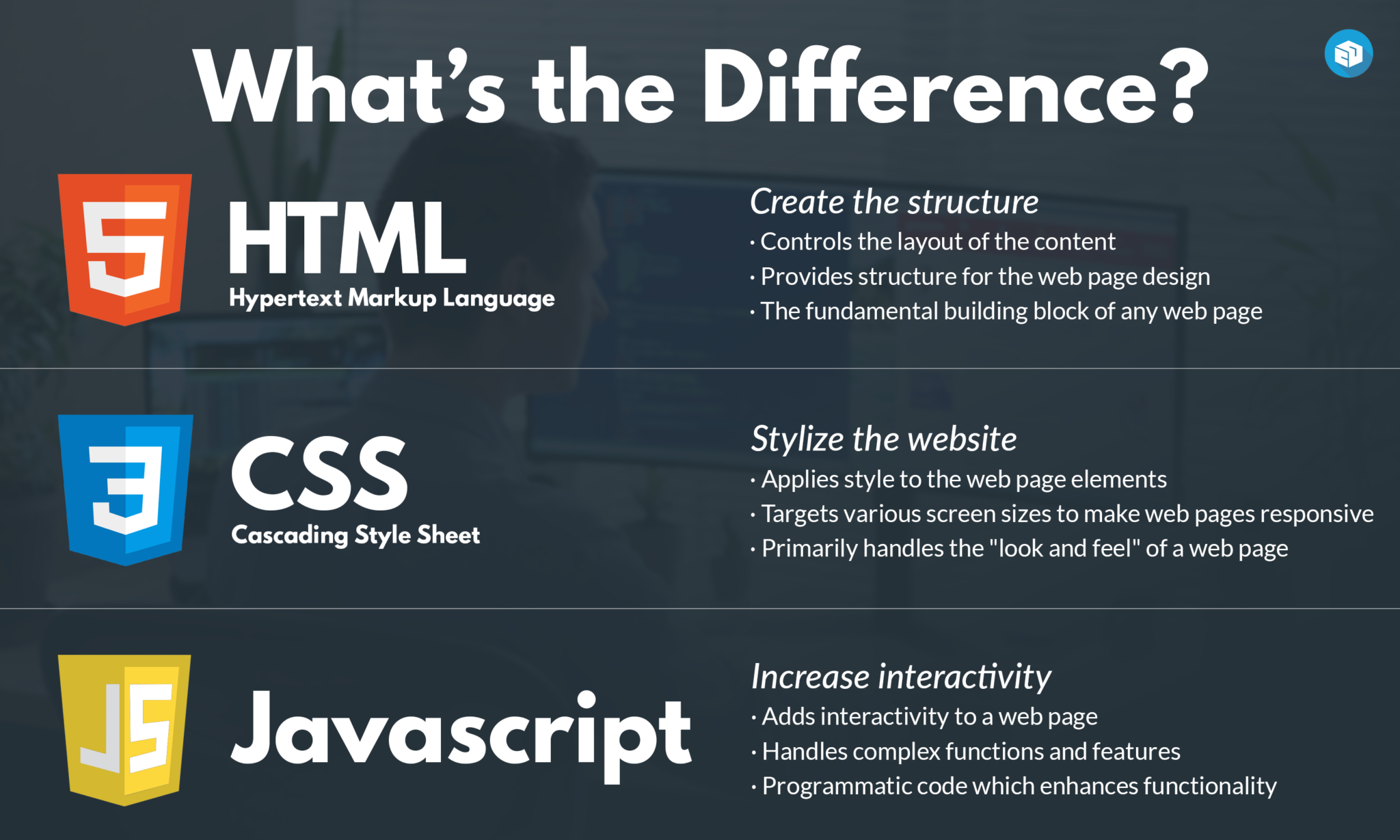
HTML is responsible for structure, CSS for style.
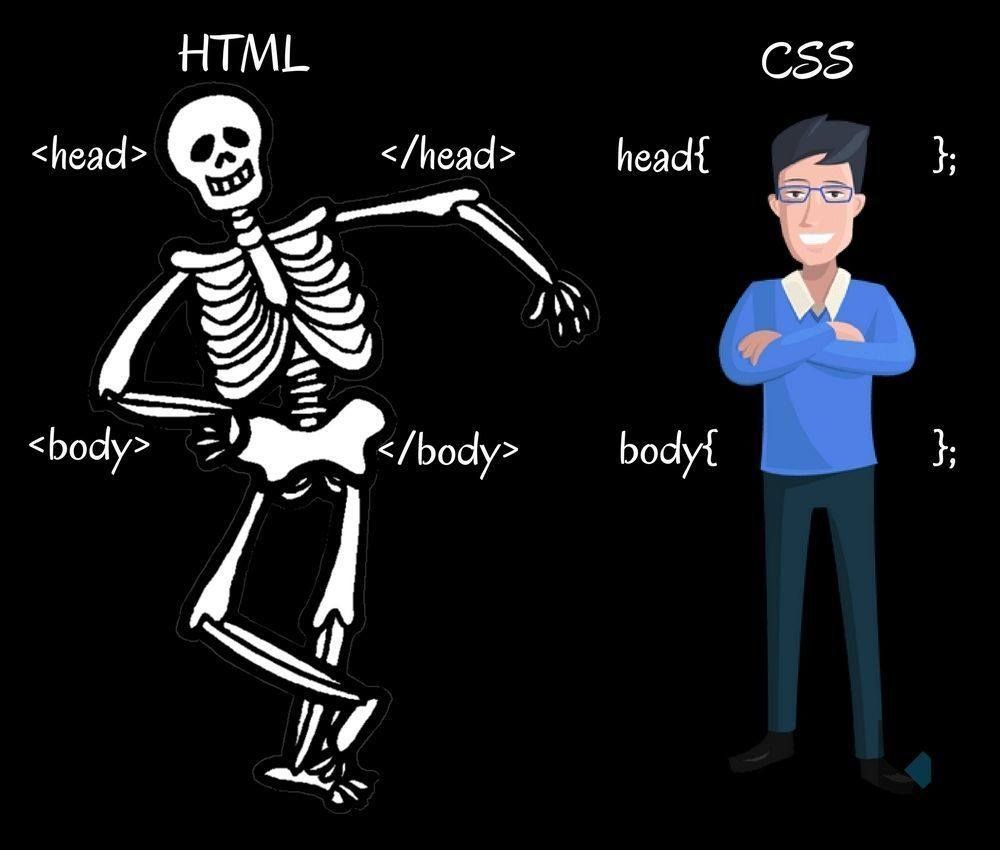
HTML stands for Hyper Text Markup Language. CSS - Cascading Style Sheets.
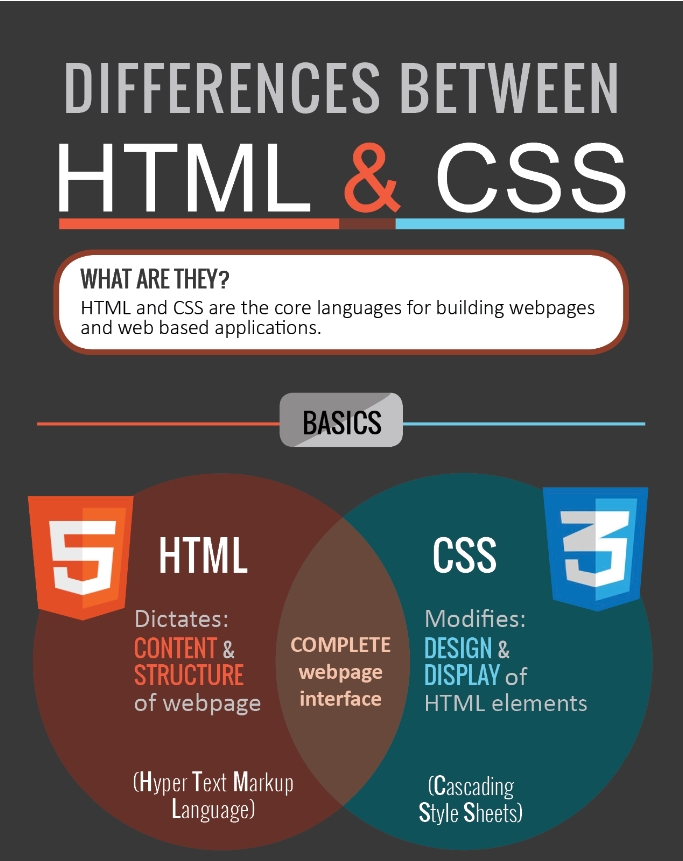
The purpose of HTML is to publish online docs. CSS - to design.
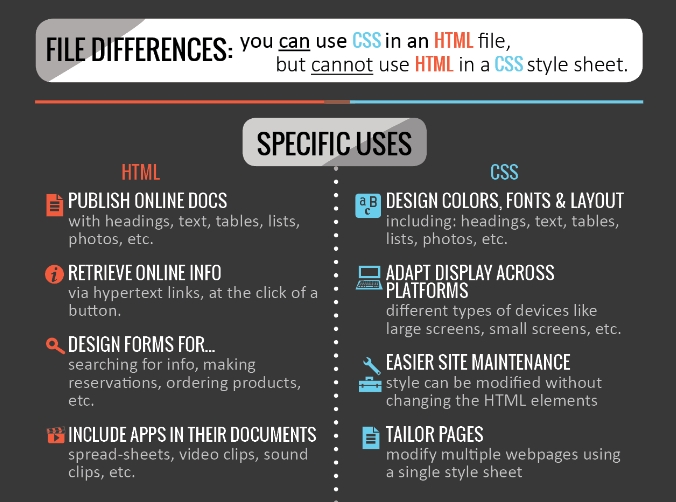
HTML consists of tags. CSS consists of selectors.
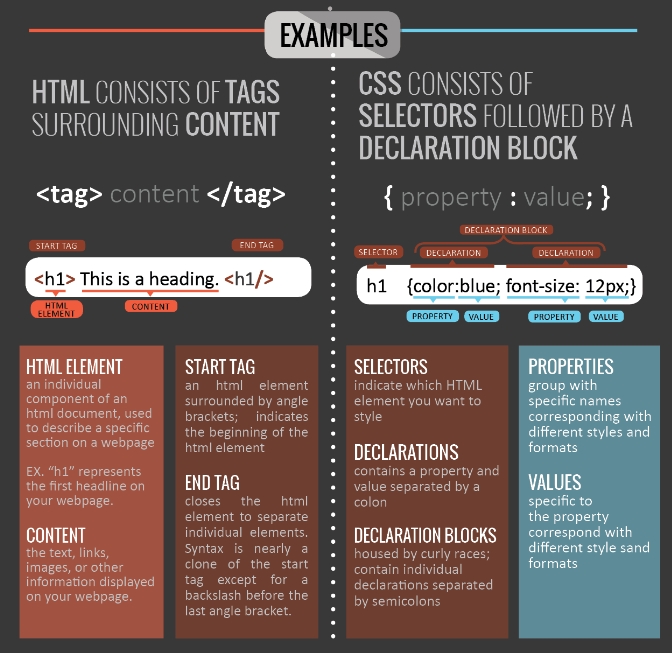
But the general difference looks in the following way:
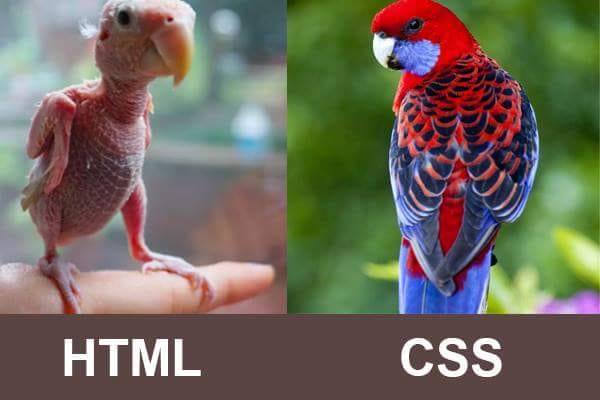